this keyword in java
There can be a lot of usage of java this keyword. In java, this is areference variable that refers to the current object.
Usage of java this keyword
Here is given the 6 usage of java this keyword.
- this keyword can be used to refer current class instance variable.
- this() can be used to invoke current class constructor.
- this keyword can be used to invoke current class method (implicitly)
- this can be passed as an argument in the method call.
- this can be passed as argument in the constructor call.
- this keyword can also be used to return the current class instance.
Suggestion: If you are beginner to java, lookup only two usage of this keyword.
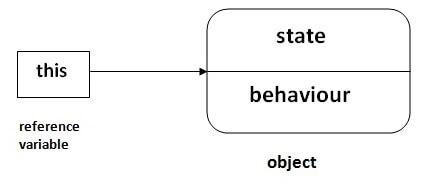
1) The this keyword can be used to refer current class instance variable.
If there is ambiguity between the instance variable and parameter, this keyword resolves the problem of ambiguity. |
Understanding the problem without this keyword
Let's understand the problem if we don't use this keyword by the example given below: |
- class Student10{
String name;
student(int id,String name){
id = id;
name = name;
}
void display(){System.out.println(id+" "+name);}
public static void main(String args[]){
Student10 s1 = new Student10(111,"Karan");
Student10 s2 = new Student10(321,"Aryan");
s1.display();
s2.display();
}
}
Output:0 null 0 null
In the above example, parameter (formal arguments) and instance variables are same that is why we are using this keyword to distinguish between local variable and instance variable. |
Solution of the above problem by this keyword
//example of this keyword
class Student11{
int id;
String name;
Student11(int id,String name){
this.id = id;
this.name = name;
}
void display(){System.out.println(id+" "+name);}
public static void main(String args[]){
Student11 s1 = new Student11(111,"Karan");
Student11 s2 = new Student11(222,"Aryan");
s1.display();
s2.display();
}
}
Output111 Karan 222 Aryan
If local variables(formal arguments) and instance variables are different, there is no need to use this keyword like in the following program: |
Program where this keyword is not required
class Student12{
int id;
String name;
Student12(int i,String n){
id = i;
name = n;
}
void display(){System.out.println(id+" "+name);}
public static void main(String args[]){
Student12 e1 = new Student12(111,"karan");
Student12 e2 = new Student12(222,"Aryan");
e1.display();
e2.display();
}
}
Output:111 Karan 222 Aryan
2) this() can be used to invoked current class constructor.
The this() constructor call can be used to invoke the current class//Program of this() constructor call (constructor chaining) class Student13{ int id; String name; Student13(){System.out.println("default constructor is invoked");} Student13(int id,String name){ this ();//it is used to invoked current class constructor. this.id = id; this.name = name; } void display(){System.out.println(id+" "+name);} public static void main(String args[]){ Student13 e1 = new Student13(111,"karan"); Student13 e2 = new Student13(222,"Aryan"); e1.display(); e2.display(); } }
constructor (constructor chaining). This approach is better
if you have many constructors in the class and
want to reuse that constructor.
Output: default constructor is invoked default constructor is invoked 111 Karan 222 Aryan
Where to use this() constructor call?
No comments:
Post a Comment